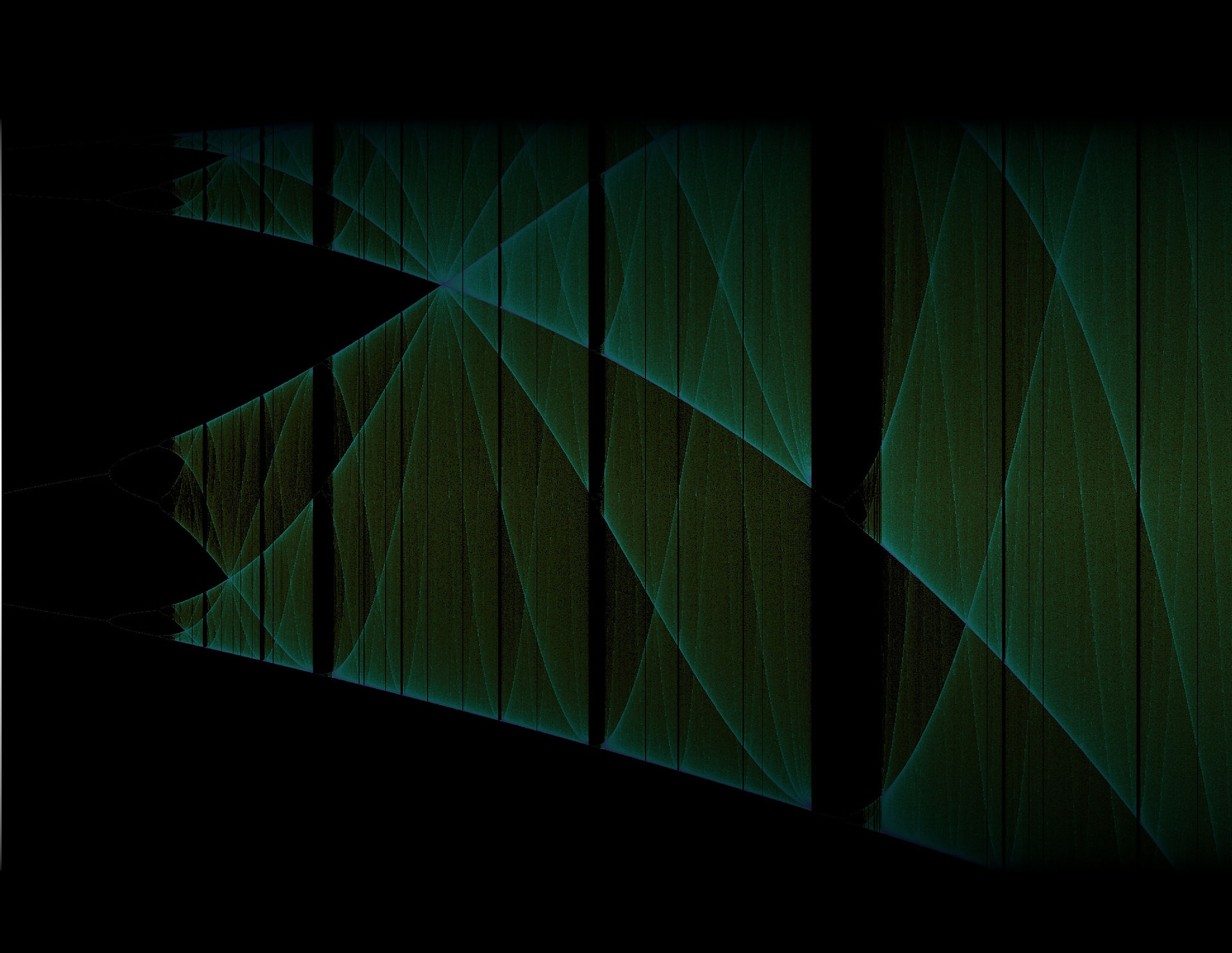
To automate the process, I've learned just enough Python to recreate his Collatz conjecture calculator. It even counts the reiterations. Try it out ↓
EUCLID & PYTHON
VERSUS
THE COLLATZ CONJECTURE
As a bit of background: I don't have a full degree in math. I am only an enthusiast. But my cousin did. In fact, he used to teach calculus at the University of Michigan, and has moved up from there. He was a student of Professor Jeffrey Lagarias, who famously spent a lot of time on this easy to explain, but impossible to prove, math conjecture.
​
My cousin and I once sat down at a local tavern, had a few beers, and discussed the Collatz conjecture. In particular, I had a suspicion about its possible... cousins. Entertaining me, he wrote and ran a few Python scripts on his phone to test my idea out. As a result of this, I began learning Python, and how to re-create his calculators.
​
We came to a startling conclusion, that I haven't seen anyone else talk about. Even on the OEIS. I haven't read through Lagarias's book yet. It's likely he, or someone else who tread this path, noticed this phenomenon as well. Still, I thought it good to communicate this extended idealization of the Collatz conjecture. An idea that my cousin, and his code, helped me justify exploring more deeply.​
​
If you don't know, the Collatz conjecture, or the 3N+1 problem, says that if you do as said in this xkcd comic, and repeat the process, ignoring the dire consequences, the sequence always reaches 1.
​
7, for example, is odd. So the first step is 7*3+1=22. Then 22 is even, so the second step is 22/2 = 11. Repeat the process, you get 7, 22, 11, 34, 17, 52, 26, 13, 40, 20, 10, 5, 16, 8, 4, 2, and finally 1. Then 1 loops through 4, 2, 1... forever.
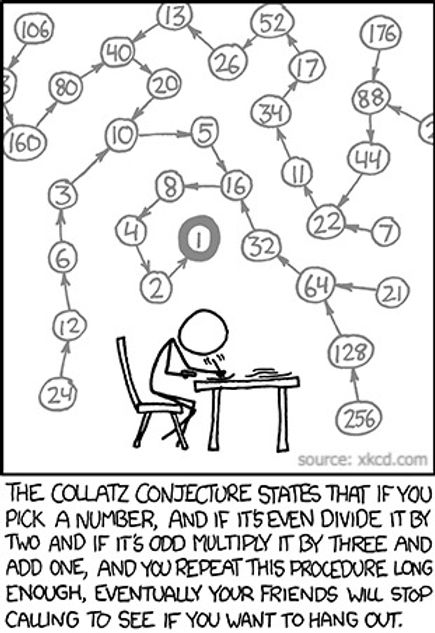
(and beer)
There is courage in hopelessness.
-Slavoj Žižek
To automate the process, I've learned just enough Python to recreate his Collatz conjecture calculator. It even counts the reiterations.
Try it out→
[Note: Assume I always mean positive integers. If it does nothing, or otherwise messes up, hit the "â–º" button to reset the console]
A motivating sidebar: Euclid's proof of infinite primes
If you're familiar with Euclid's classical proof that there are infinitely many primes, I encourage you to pause and think about where I was going with this, before you continue reading...
​
If not, here's a summary:
-
Any whole number can be expressed as a multiple of primes (except 1)
-
Suppose we've generated an exhaustive list of every prime we had access to; P1 (2), P2 (3), P3 (5), P4 (7), and so on, up to PN (the largest known prime)
-
Given the definition of a prime, which is any whole number that is not divisible by another whole number, PN cannot be the largest possible prime, because...
-
There will always be some larger number that is the product of all the known primes. If you add or subtract 1, that new number cannot be divisible by anything on that list, making it coprime (non-divisible) to all the listed primes.
That means even if (P1 * P2 *...* PN) + 1 itself isn't prime, none of its prime factors will appear on the list of {P1, P2, ..., PN}.
For example, ( 2 * 3 * 5 * 7 * 11 * 13 ) + 1 = 30,031
30,031 factors into two primes, 59 × 509
These new primes are larger than all the prime factors of 30,030.
This fact always holds true for listed consecutive primes.
But what does the 3N+1 problem have to do with Euclid's primes?
With all this in mind, can you see any connections between Euclid's proof of infinitely many primes, and the Collatz conjecture? The most obvious is that the Collatz sequence literally involves prime numbers; either divide N by the lowest prime there is (2), or multiply it by the second smallest prime (3) and add 1. And just like we were doing with Euclid's proof, by adding 1 to any integer times 3, we guarantee the new value will always be coprime to 3. Also, by only applying this function to odd numbers, we guarantee the output will be even.
​
17 is odd, and 17*3+1=52, which is divisible by 2, but not 3.
​
It's impossible to overstate how crucial the "±1" operation is, when it comes to primes and factorization. Mersenne primes are expressible as 2^P-1, and the largest prime discovered so far, a Mersenne, is equal to 2^82,589,933−1. What a night and day difference, for such massive numbers, over such a tiny interval! From a pure power of 2, to Earth's greatest known prime, just by subtracting 1.
Yet, we can guess why that is, without diving deep into number theory. If x=y±1, it's guaranteed x and y will always be coprime to each other.
Pure powers of 2 are good places to look for large, "clean" numbers. Because naturally, pure powers of 2 don't factor into anything besides 2. Which means their ±1 neighbors are by far the most likely nearby numbers to not factor into anything, besides themselves. But it's not a guarantee. Incidentally, the 2^P-1 formulation of primes only works if P is prime, and even then, not always. 82,589,933, for example, is prime. But you won't have to keep this in mind for this article. It's just a primer to get you thinking a certain way.
What the Collatz sequences do, is cycle through numbers in a seemingly random pattern, seeded by the unpredictable behavior of primes factors. Eventually, a number in its sequence reaches a power of 2, and goes straight down to 1. But will each Collatz sequence always reach a power of 2?
​
So far, the answer seems to be yes. Go ahead and mash in as large a number as you want into that trinket Python console, it'll reach 1. And the number of iterations will almost certainly be within a few hundred.
​
Nonetheless, two counter-possibilities haven't been eliminated:
-
A number may begin a sequence that diverges off to infinity. To do so, it must continually avoid powers of 2, along with all other numbers that converge to 1 along the way.
-
A number initiates a loop, which doesn't reach 1, but does reach a number in the sequence that is either twice that of a previous term, or is one less than a third of a previous term.
Neither of these phenomena have ever been observed, at least in the 3N+1 version of this problem. We don't know that they don't. If they do happen, they must be well beyond the quintillions. But without going to such lengths, things get very interesting, very quickly, when we start playing with Collatz's rules...
An Extended Collatz Conjecture: P*N+1
Armed with Euclid's ideas when introduced to Collatz, one might imagine the 3N+1 problem as being just one member of an infinite family of related functions. Recall the alternative interpretation of the Collatz conjecture: "Either divide N by the lowest prime there is (2), or multiply it by the second lowest prime (3) and add 1." What if we repeatedly divide N by the lowest 2 primes until you couldn't, then multiply it by the next largest and add one, then repeat? Or divide by the lowest 3 primes until you can't, then multiply by the fourth, add 1, then repeat? ...And so on and so forth, ad infinitum...?
​
If these prime-extended, Collatz-like conjectures sound cumbersome to do by hand, don't worry, I've automated them with Python as well.
The rules for these two calculators are:
If N is divisible by 2, divide it by 2
If N is divisible by 3, divide it by 3
If N is coprime to 2 and 3, multiply it by 5 and add 1
For these: If N is divisible by 2, divide it by 2
If N is divisible by 3, divide it by 3
If N is divisible by 5, divide it by 5
If N is coprime to 2, 3 and 5, multiply it by 7 and add 1
Double click inside the consoles and use the keys "Home" (go to top) "page up" "page down" & "End" (go to bottom) to quickly navigate through the data.
You can also scroll with the mouse wheel.
The automatic calculators have to stop after a few thousand numbers. This website is prevented from being computationally overloaded by the unending task I've given it, which is an important security feature. But I assure you, the 5N+1 and 7N+1 functions seem to proceed in the exact same way as Collatz's original 3N+1 function.
I had to limit the check to sequences under 2000 for these online compilers. Try my code on your own desktop python console. Press the "edit" button (the pencil icon, to the left of "â–º"). It will show you the scripts running these consoles. You are free to look through, copy, edit and optimize any code on this page. Whether or not you're Python savvy, run this code on a desktop Python editor. In the automatic calculators, just change "for n in range(1999):" to "for n in range(999999999):" to test these up to 1 billion. This website alone cannot handle that strain.
However, things suddenly change when we try out the 11N+1 function. See what happens when we apply its rule set, up through the integers, in this console:
If N is divisible by 2,
divide it by 2
If N is divisible by 3,
divide it by 3
If N is divisible by 5,
divide it by 5
If N is divisible by 7,
divide it by 7
If N is coprime to 2, 3, 5 & 7,
Multiply it by 11 and add 1
The 11N+1 Function Loops at 17
17, 188, 94, 47, 518, 259, 37, 408, 204, 102, 51, 17
Proof that these prime-generalized versions of the Collatz function don't always converge to 1. When I found this, I was typing away on a calculator, so victory here doesn't quite go to the machines... at least not fully. But this doesn't say anything about whether 5N, or 7N, or most importantly, 3N ever diverge. All we've proven is that 11N does. Nonetheless, let's continue with 11N+1, and see if any more interesting patterns arise. Here's an 11N loop-detecting calculator. What new loop sequences can you find? And can you find any infinite divergences?
So, the 11N+1 conjecture's results betray the literal phrasing of the Collatz conjecture's argument. Yet does it betray the spiritual phrasing of the argument? That is, can you find any natural numbers that don't reach either 1 or 17?
​
It seems now, that we've come across a second stable looping value, that I'll refer to as a loop floor, in this extended, Collatz-like series. A set of sequences that never reach 1, but loop at some higher value. Why might this occur? Why would 3N, 5N, and 7N always seem to converge on 1, and why does this pattern change at 11N? If I had a satisfying answer, I'd likely have an answer to the famous initial question too. But here's a thought: with 3N, 5N, and 7N, the space of operations are much denser than 11N. That is, the functions within 3N, 5N, & 7N all involve numbers that are no more than 2 integers apart from one another. This means numbers in those sequences usually have an easier time reducing their sizes, than growing, versus 11N. But even though 11N has a greater variety of division operations, the sequence is still liable to grow larger than 3N, 5N, & 7N at any given point. Perhaps the larger gap between 7 and 11, compared to all the previous primes, is what causes that second loop.
So, are there more loop floors, besides 1 and 17, as we climb higher in PN+1 functions? Well, the gap between 11 and 13 is small once more, so you might think they'll have a harder time reaching numbers that don't settle on one. But the result is surprisingly close...
13N will always loop at either 1 or 19...
Double click inside the consoles and use the "Home"(go to top) "page up" "page down" & "End" keys to quickly navigate, and you can scroll with the mouse wheel
...17N always loops at 1 or 43...
...And strangely enough, 19N doesn't form a loop that avoids 1 until 46,063.
Give it a few seconds before resetting, it takes a while...
What do these loops reveal about Collatz?
This table shows the data for the PN+1 functions under 100:
Sequence
Highest known loop floor
3N:
5N:
7N:
11N:
13N:
17N:
19N:
23N:
29N:
31N:
37N:
41N:
43N:
47N:
53N:
59N:
61N:
67N:
71N:
73N:
79N:
83N:
89N:
97N:
1
1
1
17
19
43
46,063
179
*1
67
2,137
*1
174,569
859
19,559
73
97
181
306,511
409
*1
89
*1
631
Loop length
3
3
3
11
15
84
61
18
*39
128
71
*4
58
61
63
33
12
14
33
35
*11
99
*63
127
*asterisks mean a higher loop floor is expected, but is beyond my computational capacity to reach
Again, the smallest number in any P*N+1 loop is called the "floor value" for that loop. It's always the lowest number you'll find in a given PN+1 function. 1 is always the first one. Every PN+1 function listed here has been tested up to 1 million. These "highest known loop floors" seem very special. That is, they are the only numbers (besides 1) that any PN+1 sequence is guaranteed to reach. Let that sink in for a moment, and consider what it suggests about Collatz.
​
It suggest Collatz might have more to do with the nature of loops, than strictly convergence on 1. That's the biggest takeaway from this exploration. PN+1 opens the question up to further processing, with smarter algorithms, and connections to other fields of mathematics. To spitball, perhaps there's a also connection to the Hydra Problem? Which also involves sequences that explode at first, then converge to zero. This is worth investigating in the future, despite the fact that Collatz and Hydra rely on different number systems.
In future explorations, I'll also begin using sieves (like the Sieve of Eratosthenes), to remove unnecessary sequences. To use this sieve, list the numbers along the number line. Then cross out multiples of all numbers you come across as you increment by one (except 1). This leaves you with primes only. It's simple, but effective, especially for Python users. (I'm learning)
​
Let's take a moment to appreciate this data set's other features. For one, the floors don't just grow, or shrink with each P. They jump all over the place. Meaning there's no real guarantee that 7N, 5N, or even 3N don't form a loop at some huge floor value.
​For a number to avoid hitting '1' immediately, it can't be divisible by any number, up to that sequence's P-value. There's also a recurring pattern to these floor values. Like primes over 10, they all end in either a 1, 3, 7 or a 9. Why?
​
Consider this: Every prime number greater than 10 must end in a 1, 3, 7, or 9. That also means that any given product, of any number of primes (above 10), must also end in a 1, 3, 7, or 9. The Sieve of Eratosthenes rules out larger primes ending in any other numbers, and their multiples on each other, too. Try it for yourself, if you're unfamiliar with this fact. This means inputs to PN+1 operations guarantee their outputs are coprime to all numbers below that P. Remember Euclid?
​
On a semi-related note: as a consequence of reiteration, every 4th power of any number ending in 1, 3, 7, or 9 ends in a 1 (3^4=1, 3^8=81, 3^12=6561).
​
For 11N and beyond, any given loop floor is guaranteed to not be divisible by 2 or 5, and so must end in 1, 3 , 7, or 9. To find even larger loop floors, we may use different base number systems to make the search space more amenable. But this is only useful for the functions greater than 7N.
​
For the 3N and 5N conjectures, one may consider using a smaller base number systems, like base 6 and binary, instead of traditional base 10. Just remember, on its own, the only thing this reveals is that the best place to look for loops in 3N+1 is along the odd numbers. Which should be obvious, but it's a great tip for naïve programmers looking to reduce data waste in future projects.
Let's take one last automated look at these loop floors. Graphically this time.
So far, it seems to be the case that there are only two Collatz "trees" for any PN+1"forest". These trees represent the sequences for all whole numbers that reach 2 specific values. The python calculators above will continue printing sequences, until told to stop at a value that I arbitrarily decided, or if they loop on any number. But these sequences never break naturally, even after billions of sequences tested. Likewise, this forest graphic should also reach every whole number.
Here's a visualization of an 11N+1 Collatz "forest", generously rendered by Tucan, check out their Github!
Notice the two trees: one with numbers that only loop at 1 (pink), and numbers that only loop at 17 (blue)
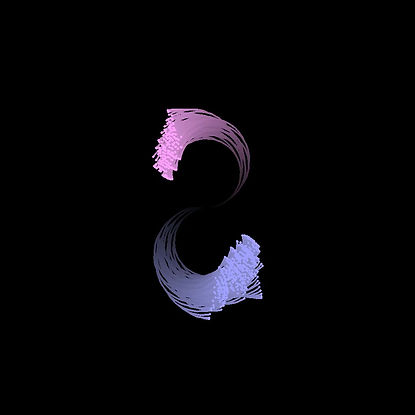
All whole positive numbers will either reach 1 or 17 in the "11N+1, or divide N by all the primes up to 7" function.
Every PN+1 sequence so far behaves similarly, in having only 2 trees. For artistic, illustrative purposes, these two disconnected sets of sequences are offset by 180° to each other.
Usefulness, Future Explorations, and Closing Thoughts
With the PN+1 conjecture in hand, we can start exploring the properties of stable, but unpredictably located loops, in Collatz conjecture-like setups. I've overseen billions of these automated sequences so far, with this naive approach. Although it is thus far a limited exploration, it suggests something new about the character of any potential loops in the Collatz conjecture.
​
It now seems possible that there is only 1 floor value, beyond 1, at which a Collatz loop can form (if any).
Granted, this is my ad-hoc hypothesis after a very computationally limited trial. I've only checked for secondary floor values up to one billion. Considering the orders of magnitude involved so far, it could very well be that there are countless floor values for all sequences, increasingly spaced apart, way out in the Googolplexes and beyond. I'm not able to check that far, but on the other hand, I've found no exceptions to this hypothesis, after a good part of a trillion equations have been computed.
This blog post raises a new question, that must be explored in multi-part series when I have more time and have compiled more facts. Deadlines are deadlines, after all, and this was meant to be a 10 minute entry. Many discoveries in this post were made along the way, in the process of writing and testing the scripts for this article. More are sure to follow.
​
Can we find more than 2 loops for any given P? If not, then we may be looking at a situation where only one other loop floor (besides 1) is possible in the Collatz conjecture. Keep in mind, reiterative loops are structures that haven't yet yielded interesting results on 3N alone. This angle may offer helpful clues to find otherwise unobservable behaviors of contradicting conditions, since 3N always tends towards 1, at least on our limited computers.
What about 5N and 7N? I feel semi-comfortable assuming 29N and 41N reach non-1 loop floors, evidently in the billions or beyond. Yet do 5N and 7N have non-1 loop floors? Or do they behave more like the original 3N+1 problem?
​
Before I close, let's explore one quick thought experiment with PN+1 on the other contradictory condition. Can we create a similar sequence that explodes off to infinity? Sure you can! Just remove all the division operations!
​
In all seriousness, selectively removing division operations leads to interesting properties when applied to the problem. Numbers in these "partially filled" PN+1 functions behave like their counterparts in the "filled" functions. But it was far easier to illustrate this idea, if the sequences involved easily ran down to 1. That is, with a "filled" sequence. What I mean by this, is if you remove the N/3 operation in the 5N+1 function, all the numbers still seem to reach 1, or some other loop floor. But they take much longer to do so. Look how the 5N+1 function behaves without N/3, and see what I mean:
Click to enlarge
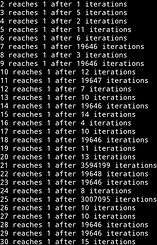
This is a property I'll have to explore in depth, later on in this series. This additional fact, suggests that even all the "unfilled" sequences, eventually reach 1, or loop above it. Suppose we took 97N+1, and stipulated that we could only divide it by two if it was even, or multiply it by 97 and add 1 if it's odd. You may not be able to easily solve any of these sequences, even with computers automating the process. But it may be, that even something as outlandish as {97N+1 or N/2} always converges to 1, or at least, some other finite loop floor. What do you think? Am I barking up the right Collatz tree? Reach out to me on Discord, if you have something interesting to add to this discussion.
​
What simultaneously makes the Collatz conjecture so pernicious, AND so inspiring, is that it offers a tantalizing hint at the final behaviors of chaotic seeming systems. Behaviors after they've gone through an infinite number of quasi-random math operations. If a connection is discovered, the implications could be phenomenal. Powerful, new cryptographic engines might be created from the idea, especially if loops floors can be provably located. Yet, because of the prime-factorization seeded, chaotic nature of these arbitrarily bounded sequences, we have great difficulty reconciling their final behaviors.
Collatz exists in conflict. It has some calculus-like spirit of a smooth predictability after infinite operations, and it has a fractal geometry-like spirit that adds more jaggedness, more complexity, the further an idea is carried out. But even if tackling the Collatz conjecture can make you feel like a dog chasing their own tail, it's still possible to blindly wander somewhere interesting doing so.
​
With that, if there really is at most one Collatz loop above 1, I'll leave you with the iconic line from Tolkien's The Lord of the Rings:
One Ring to rule them all,
One Ring to find them,
One Ring to bring them all,
And in the Darkness, bind them.
Thank you(s)!!
Thanks for reading this! Big thank you to Grant Sanderson at 3Blue1Brown for prompting the Summer of Math Exposition!
At the risk of 3Blue1Brown-nosing, I'd just like to say, I've been watching Grant's Sanderson's channel since before he hit 100k subscribers, and I'm proud to see it skyrocketing up to almost 4M subs! 3Blue1Brown is my favorite math channel on Youtube, and I'm sure a ton of poeple can relate. I knew this style of visual demonstrations of math would catch on, and I'd be happy to emulate this success if I can, if nothing else than for new knowledge to be spread. I'm trying out something I might have never done before, but have been thinking about doing for quite a while. And my aim for these blog posts will be an increasing use of programmatic interactivity, simplicity in explanation, and visual demonstrations.
And a HUGE thank you to all the people on Discord (particularly Tucan, and VictorSP) who helped me clarify my math, write better scripts, and add amazing visuals! You carried my work when my brain burnt out, and gave me things I could not have had by the deadline alone. Second to lastly to my cousin, who showed me Python as a viable toolset for this problem, and years of math insights I would've only found through university. Yes, I'm very green with Python. But I hope to do some even more interesting work with this incredible computational device that's now opening up to me. And lastly, to my girlfriend, who was patient enough to hear me ramble on for hours while I was stuck in a loop, and smart enough provide genuine insights for clarifying this, even if she doesn't think of herself as a math person. And if all goes well, I'm looking forward to launching a YouTube channel assosciated with this blog. Thanks everyone!